This post was published in 2022-02-16. Obviously, expired content is less useful to users if it has already pasted its expiration date.
Table of Contents
补充一些Numpy的用法 *
复习之前写过的Numpy数组操作内容:Numpy数组操作(reshape, concatenate, vstack 等) 🔗 [2021-11-02 - Truxton's blog] https://truxton2blog.com/2021-11-02/
Numpy hstack
(本程序由上次写的程序继承拓展而来)
import numpy as np
a = np.arange(1, 9, 1).reshape(4, 2)
print('a:')
print(a)
print('\n')
b = np.arange(-1, -9, -1)
b = b.reshape(4, 2)
print('b:')
print(b)
print('\n')
print('np.vstack((a,b))')
print(np.vstack((a, b)))
print('\n')
print('np.concatenate((a,b),axis=0)')
print(np.concatenate((a, b), axis=0))
print('\n')
print('np.concatenate((a,b),axis=1)')
print(np.concatenate((a, b), axis=1))
print('\n')
print('np.hstack(a,b)')
print(np.hstack((a, b)))
结果:
a:
[[1 2]
[3 4]
[5 6]
[7 8]]
b:
[[-1 -2]
[-3 -4]
[-5 -6]
[-7 -8]]
np.vstack((a,b))
[[ 1 2]
[ 3 4]
[ 5 6]
[ 7 8]
[-1 -2]
[-3 -4]
[-5 -6]
[-7 -8]]
np.concatenate((a,b),axis=0)
[[ 1 2]
[ 3 4]
[ 5 6]
[ 7 8]
[-1 -2]
[-3 -4]
[-5 -6]
[-7 -8]]
np.concatenate((a,b),axis=1)
[[ 1 2 -1 -2]
[ 3 4 -3 -4]
[ 5 6 -5 -6]
[ 7 8 -7 -8]]
np.hstack(a,b)
[[ 1 2 -1 -2]
[ 3 4 -3 -4]
[ 5 6 -5 -6]
[ 7 8 -7 -8]]
Numpy reshape
import numpy as np
a = np.arange(1, 9, 1).reshape(4, 2)
print('a:')
print(a)
print('\n')
print('a.reshape(-1, 1)')
print(a.reshape(-1, 1))
print('\n')
print('a.reshape(1,-1)')
print(a.reshape(1, -1))
print('\n')
print('a.reshape(-1)')
print(a.reshape(-1))
# 下面的代码会报错:ValueError: cannot reshape array of size 8 into shape (1,)
# print('\n')
# print('a.reshape(1)')
# print(a.reshape(1))
print('\n')
print('a.reshape(8)')
print(a.reshape(8))
结果:
a:
[[1 2]
[3 4]
[5 6]
[7 8]]
a.reshape(-1, 1)
[[1]
[2]
[3]
[4]
[5]
[6]
[7]
[8]]
a.reshape(1,-1)
[[1 2 3 4 5 6 7 8]]
a.reshape(-1)
[1 2 3 4 5 6 7 8]
a.reshape(8)
[1 2 3 4 5 6 7 8]
Numpy flattern
import numpy as np
a = np.arange(1, 21, 1).reshape(4, 5)
print('a:')
print(a)
print('\n')
print('a.flatten()')
print(a.flatten())
结果:
a:
[[ 1 2 3 4 5]
[ 6 7 8 9 10]
[11 12 13 14 15]
[16 17 18 19 20]]
a.flatten()
[[ 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20]]
np.where()
In [1]: a = np.arange(1, 21, 1).reshape(4, 5)
In [2]: a
Out[2]:
array([[ 1, 2, 3, 4, 5],
[ 6, 7, 8, 9, 10],
[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20]])
In [3]: b = a.copy()
In [4]: b[np.where(b < 10)] = -1
In [5]: b
Out[5]:
array([[-1, -1, -1, -1, -1],
[-1, -1, -1, -1, 10],
[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20]])
In [6]: np.where(a < 10, 999, a)
Out[6]:
array([[999, 999, 999, 999, 999],
[999, 999, 999, 999, 10],
[ 11, 12, 13, 14, 15],
[ 16, 17, 18, 19, 20]])
In [7]: np.where(1, 999, a)
Out[7]:
array([[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999]])
In [8]: np.where(-1, 999, a)
Out[8]:
array([[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999]])
In [9]: np.where(0.1, 999, a)
Out[9]:
array([[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999],
[999, 999, 999, 999, 999]])
In [10]: np.where(0, 999, a)
Out[10]:
array([[ 1, 2, 3, 4, 5],
[ 6, 7, 8, 9, 10],
[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20]])
复习或学习一些数字图像处理方法
Thresholding
🔗 [OpenCV: Image Thresholding] https://docs.opencv.org/4.x/d7/d4d/tutorial_py_thresholding.html
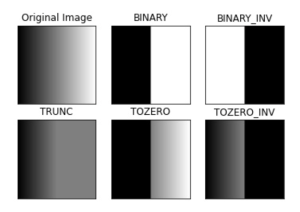
Filtering
比如高斯模糊
🔗 [OpenCV: Smoothing Images] https://docs.opencv.org/3.4/d4/d13/tutorial_py_filtering.html
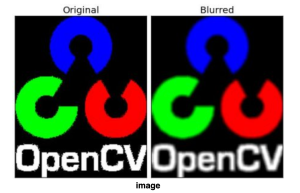
轮廓提取算法(参考cv2.findContours)
🔗 [python - Detect white rectangle on black & white image and crop (OpenCV) - Stack Overflow] https://stackoverflow.com/questions/60794387/detect-white-rectangle-on-black-white-image-and-crop-opencv
🔗 [OpenCV轮廓提取算法详解findContours() - 知乎] https://zhuanlan.zhihu.com/p/107257870
🔗 [图像的分割之轮廓提取算法ReWz的博客-CSDN博客轮廓提取算法] https://blog.csdn.net/qq_43409114/article/details/104673115
Last Modified in 2022-02-26