This post was published in 2022-02-23. Obviously, expired content is less useful to users if it has already pasted its expiration date.
Table of Contents
搞点以前没用过的python小知识
在此之前可能需要复习:🔗 [2021-10-19 - Truxton's blog] https://truxton2blog.com/2021-10-19/
格式化输出(部分)
🔗 [Python3 print 函数用法总结 | 菜鸟教程] https://www.runoob.com/w3cnote/python3-print-func-b.html
str = "the length of '%s' is %d" % ('numpy', len('numpy'))
print(str)
print("the length of '%s' is %d" % ('numpy', len('numpy')))
######################
for i in range(0, 3):
print(i, end=' ')
the length of 'numpy' is 5
the length of 'numpy' is 5
0 1 2
注意上面的输出结果没有最后的空行,因为print()默认的换行符改成了空格。
python built-in data types
🔗 [Python Data Types] https://www.w3schools.com/python/python_datatypes.asp
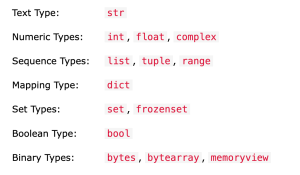
(怎么又忘了)python set, tuple, list
上一次复习:🔗 [2021-10-19 - Truxton's blog] https://truxton2blog.com/2021-10-19/
老图:
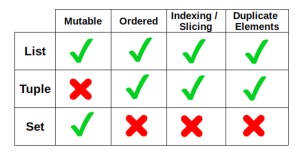
自从上次复习到现在,我写的代码里一直没用过set,这是非常愚蠢的(我甚至用dictionary key:None)
a = {1, 2, 3, 3, 3, 3, 3, 3, 4, 1.1, float(1.0), 1.0}
print(a)
运行结果:
{1, 2, 3, 4, 1.1}
原因:
🔗 [dictionary - True and 1 and 1.0 evaluated to be same in python dictionaries - Stack Overflow] https://stackoverflow.com/questions/52402133/true-and-1-and-1-0-evaluated-to-be-same-in-python-dictionaries

escape sequence
🔗 [Escape Characters — Python Reference (The Right Way) 0.1 documentation] https://python-reference.readthedocs.io/en/latest/docs/str/escapes.html
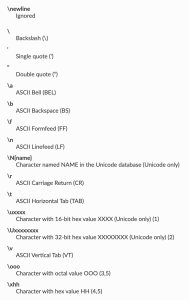
pure function和side effect
解释的很烂 🔗 [Functional Programming HOWTO — Python 3.10.2 documentation] https://docs.python.org/3/howto/functional.html
🔗 [副作用 (计算机科学) - 维基百科,自由的百科全书] https://zh.wikipedia.org/zh-hans/副作用_(计算机科学)
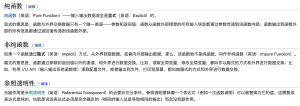
后续阅读:🔗 [函数式编程的Python实践(1):本质 - 知乎] https://zhuanlan.zhihu.com/p/88198966
function和method
method是一种特殊的function,它和object绑定:
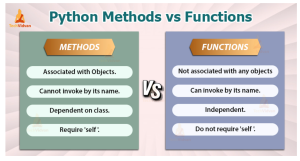
🔗 [The Difference Between a Function and a Method - Codingem] https://www.codingem.com/python-what-is-the-difference-between-a-function-and-a-method/
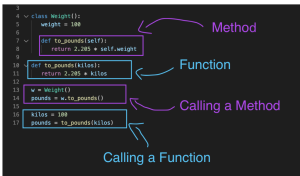
sequence data type
🔗 [What is a sequence data type in Python?] https://www.tutorialspoint.com/What-is-a-sequence-data-type-in-Python
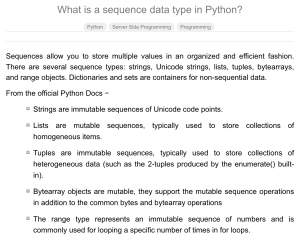
OCR辅助查找工具
Text Type: strNumeric Types: int, float, comp lexSequence Types: List, tuple, rangeMapping Type: dictSet Types: set, frozensetBoolean Type: boolBinary Types: bytes, bytearray, memoryviewthe hash of the 3 items 1, 1.0, True is the same (it equals 1). that’s what python uses as the key for dictionaries f there are no collisions. and as 1 == 1.0 == True is also True there are no collisions.Inewline IgnoredBackslash (VSingle quote ()10Double quote (“)a ASCII Bell (BEL)1b ASCIl Backspace (BS) f ASCIl Formfeed (FF)In ASCll Linefeed (LF) Niname] Character named NAME in the Unicode database (Unicode only)Lr ASCIl Carriage Return (CR)t ASCll Horizontal Tab (TAB)Luxxxx Character with 16-bit hex value XXXX (Unicode only) (1) 1UxXxxxxxx Character with 32-bit hex value XXXXXXXX (Unicode only) (2)Iv ASClI Vertical Tab (VT)1ooo Character with octal value Ooo (3,5) Lxhh Character with hex value HH (4,5)纯函数〔编辑〕纯函数(英语:Pure Function)—输入输出数据流全是显式(英语:Explicit)的。显式的意思是,函数与外界交换数据只有一个唯一渠道——参数和返回值;函数从区数外部接受的所有输入信息都通过参数传递到该西数内部;函数输出到函数外部的所有信息都通过返回值传递到该函数外部。非纯函数[编辑〕如果一个函数通过隐式(英语:Implicit)方式,从外界获取数据,或者向外部输出数据,那么,该函数就不是纯函数,叫作非纯函数(英语:Impure Function)隐式的意思是,函数通过参数和返回值以外的渠道,和外界进行数据交换。比如,读取全局变量,修改全局变量,都叫作以隐式的方式和外界进行数据交换;比如,利用 /O API (输入输出系统函数库)读取配置文件,或者输出到文件,打印到屏幕,都叫做隐式的方式和外界进行数据交换。参照透明性〔编辑〕无副作用是参照透明性(英语:Referential Transparent)的必要非充分条件。参照透明意味着一个表达式(例如一次函数调用)可以被替换为它的值。这需要该表达式是纯的,也就是说该表达式必须是完全确定的(相同的输入总是导致相同的输出)而且没有副作用。TechVidvan Python Methods vs FunctionsMETHODS FUNCTIONSAssociated with Objects. Not associated with any objectsCannot invoke by its name. Vs Can invoke by its name.Dependent on class. Independent. Meviduaniduan Require’self! Do not require ‘self:3V class Weight():C weight二100 Method OO、 def to_pounds (self): return 2.205 * self.weight卡长卡上品def to_pounds(kilos): return 2.205 * kilos Functionw = Weight()15 pounds w. to_pounds()16 kilos = 100 17 pounds = to_pounds (kilos) Calling a MethodCalling a FunctionWhat is a sequence data type in Python?Python Server Side Programming ProgrammingSequences allow you to store multiple values in an organized and efficient fashion. There are several sequence types: strings, Unicode strings, lists, tuples, bytearrays, and range objects. Dictionaries and sets are containers for non-sequential data.From the official Python Docs日Strings are immutable sequences of Unicode code points.日Lists are mutable sequences, typically used to store collections of homogeneous items.日Tuples are immutable sequences, typically used to store collections of heterogeneous data (such as the 2-tuples produced by the enumerate() built- in).日Bytearray objects are mutable, they support the mutable sequence operations in addition to the common bytes and bytearray operations日The range type represents an immutable sequence of numbers and is commonly used for looping a specific number a of times in for loops.Mutable Ordered Indexing Duplicate Slicing ElementsListTupleSet ××X