This post was published in 2021-09-25. Obviously, expired content is less useful to users if it has already pasted its expiration date.
主要内容:Python基础知识/基础语法;算法:事件/空间复杂度
python idiom
python *args , **kwargs
类似这样的:
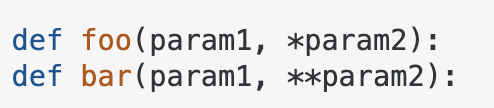
解释:"The single asterisk form (*args
) is used to pass a non-keyworded, variable-length argument list, and the double asterisk form is used to pass a keyworded, variable-length argument list." (来自https://www.saltycrane.com/blog/2008/01/how-to-use-args-and-kwargs-in-python/)
一些简单的例子帮助快速入门,见https://stackoverflow.com/questions/36901/what-does-double-star-asterisk-and-star-asterisk-do-for-parameters
(针对上面的stackoverflow链接)一些补充代码:
def bar(**kwargs):
for a in kwargs:
print(a, kwargs[a])
bar(name='one', age=27)
# name one
# age 27
========================================================
def bar(**kwargs):
for a in kwargs:
print(a, kwargs[a])
dict = {'name': 'one', 'age': 27}
bar(dict)
# TypeError: bar() takes 0 positional arguments but 1 was given
========================================================
def bar(**kwargs):
for a in kwargs:
print(a, kwargs[a])
dict = {'name': 'one', 'age': 27}
bar(name='one', age=27, dict=dict)
# name one
# age 27
# dict {'name': 'one', 'age': 27}
========================================================
def bar(*x, **y):
for item in x:
print(item)
for item in y:
print(item + ':' + y[item])
bar(1, 2, 3, 4, name='student', age='24')
# 1
# 2
# 3
# 4
# name:student
# age:24
======================================================
def bar(*x, **y):
for item in x:
print(item)
for item in y:
print(item + ':' + y[item])
bar((1, 2, 3, 4), name='student', age='24')
# (1, 2, 3, 4)
# name:student
# age:24
======================================================
def bar(*x, **y):
for item in x:
print(item)
for item in y:
print(item + ':' + y[item])
bar(1, 2, 3, 4, name='student', age='24', 5, 6)
# bar(1, 2, 3, 4, name='student', age='24', 5, 6)
# ^
# SyntaxError: positional argument follows keyword argument
此外,这个链接:https://www.saltycrane.com/blog/2008/01/how-to-use-args-and-kwargs-in-python/ 也列举了一些*args和**kwargs的代码。
如果运行下面的3个代码片段:
def bar(*x, **y):
for item in x:
print(item)
for item in y:
print(item + ':' + y[item])
l = [1, 2, 3, 4]
bar(l, name='student', age='24')
# [1, 2, 3, 4]
# name:student
# age:24
def bar(*x, **y):
def printx(x_x):
for i in x_x: print(i)
printx(*x)
for item in y:
print(item + ':' + y[item])
l = [1, 2, 3, 4]
bar(l, name='student', age='24')
# 1
# 2
# 3
# 4
# name:student
# age:24
def bar(*x, **y):
for item in *x:
print(item)
for item in y:
print(item + ':' + y[item])
l = [1, 2, 3, 4]
bar(l, name='student', age='24')
# for item in *x:
# ^
# SyntaxError: invalid syntax
第3个代码片段会报错,那么如何把它改正确呢?
应该这么修改(修改了line 9):
def bar(*x, **y):
for item in x:
print(item)
for item in y:
print(item + ':' + y[item])
l = [1, 2, 3, 4]
bar(*l, name='student', age='24')
# 1
# 2
# 3
# 4
# name:student
# age:24
python lambda : map function
list1 = [1, 2, 3, 4, 5]
list1_r = list(map(lambda item: item, list1))
print(list1_r)
# 2.283333333333333
list1 = [1, 2, 3, 4, 5]
list1_r = sum(map(lambda item: item, list1))
print(list1_r)
# 15
list1 = [1, 2, 3, 4, 5]
list1_r = list(map(lambda item: 1 / item, list1))
print(list1_r)
# [1.0, 0.5, 0.3333333333333333, 0.25, 0.2]
python判断string是否为数字:(比如'1234': yes和'1234k': no)
https://stackoverflow.com/questions/354038/how-do-i-check-if-a-string-is-a-number-float
python console I/O
代码举例:
nargs参数的含义与作用:https://stackoverflow.com/questions/20165843/argparse-how-to-handle-variable-number-of-arguments-nargs
python overload
给定一组统计数据(比如运行时间),如何衡量这组数据“很稳定”(浮动不大)?
使用Coefficient of variation(CV),见:https://en.wikipedia.org/wiki/Coefficient_of_variation
[mathjax-d]\displaylines{ CV=\frac{\sigma}{\mu} \\ \sigma: \text{standard deviation} \\ \mu: \text{mean} }[/mathjax-d]