This post was published in 2022-01-24. Obviously, expired content is less useful to users if it has already pasted its expiration date.
Table of Contents
继续复习C语言基础知识
结构体
首先是两种访问结构体成员的方式:🔗 [c - Difference between -> and . in a struct? - Stack Overflow] https://stackoverflow.com/questions/5998599/difference-between-and-in-a-struct
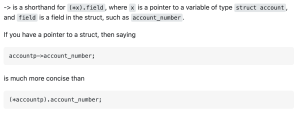
相关代码:
#include<stdio.h>
struct {
char *apache_family;
char *nginx_family;
} webserver = {"litespeed", "openresty"};
int main(int argc, char const *argv[]) {
printf("%s %s\n", webserver.apache_family, webserver.nginx_family);
printf("%s %s\n", (&webserver)->apache_family, (&webserver)->nginx_family);
printf("%s %s\n", (*&webserver).apache_family, (*&webserver).nginx_family);
return 0;
}
结果:
litespeed openresty
litespeed openresty
litespeed openresty
#include<stdio.h>
#include <stdlib.h>
struct http_family {
char *http1;
char *http2;
char *http3;
};
struct {
char *apache_family;
char *nginx_family;
} webserver = {"litespeed", "openresty"};
int main(int argc, char const *argv[]) {
printf("%s\n%s\n", webserver.apache_family, webserver.nginx_family);
struct http_family *myhttp = (struct http_family *) malloc(sizeof(struct http_family) * 1);
myhttp->http1 = "http1.1";
myhttp->http2 = "http2.0";
myhttp->http3 = "QUIC";
printf("%s\n%s\n%s\n", myhttp->http1, myhttp->http2, myhttp->http3);
return 0;
}
结果:
litespeed
openresty
http1.1
http2.0
QUIC
为了更好理解上面程序的第10~13行结构体的定义,从上面这个程序里剥离一部分:
#include<stdio.h>
struct {
char *apache_family;
char *nginx_family;
} webserver;
int main(int argc, char const *argv[]) {
printf("%s\n%s\n", webserver.apache_family, webserver.nginx_family);
webserver.apache_family = "openlitespeed";
webserver.nginx_family = "openresty";
printf("%s\n%s\n", webserver.apache_family, webserver.nginx_family);
return 0;
}
结果如下:
(null)
(null)
openlitespeed
openresty
#include<stdio.h>
#include<string.h>
struct account {
int number;
char *name;
char boss_name[10];
};
int main(int argc, char const *argv[]) {
struct account a;
a.number = 100;
char *temp = "My Name";
a.name = temp;
strcpy(a.boss_name, "BOSS");
// // 上面这行又可以写为:
// char *temp2="BOSS";
// strcpy(a.boss_name,temp2);
printf("%d\n%s\n%s", a.number, a.name, a.boss_name);
return 0;
}
结果:
100
My Name
BOSS
int32, int, int32_t, int8, int8_t
int是基本数据类型,其他的(int32, int32_t, int8, int8_t, ...)这些都只是typedef types.
🔗 [c - Difference between int32, int, int32_t, int8 and int8_t - Stack Overflow] https://stackoverflow.com/questions/14515874/difference-between-int32-int-int32-t-int8-and-int8-t

(同链接下)另一个回答:
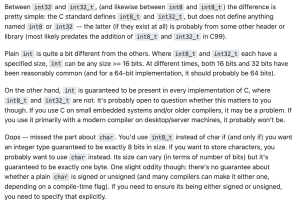
++i和i++
🔗 [C语言i++和++i的区别和用法_C语言技术网-码农有道-CSDN博客_c语言i++和++i] https://blog.csdn.net/wucz122140729/article/details/105531374
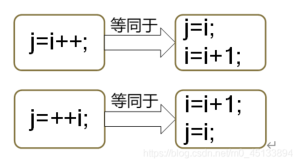
#include<stdio.h>
#include <stdlib.h>
int main(int argc, char const *argv[]) {
int i;
int j;
for (i = 0; i < 4; i++) {
printf("%d\n", i);
}
printf("\n");
for (i = 0; i < 4; ++i) {
printf("%d\n", i);
}
printf("\n");
i = 10;
j = i++;
printf("j = %d\n", j);
j = ++i;
printf("j = %d", j);
return 0;
}
结果:
0
1
2
3
0
1
2
3
j = 10
j = 12
CV: 图像卷积 通道数 池化
🔗 [CNN卷积核与通道讲解 - 知乎] https://zhuanlan.zhihu.com/p/251068800
🔗 [CNN基础知识——池化(pooling) - 知乎] https://zhuanlan.zhihu.com/p/78760534
OCR辅助查找工具
•> is a shorthand for (x) . field, where x is a pointer to a variable of type struct account and field is a field in the struct, such as account_number. If you have a pointer to a struct, then saying accountp->account_number; is much more concise than (accountp) .account_number;The _t data types are typedef types in the stdint.h header, while int is an in built fundamental data type. This make the _t available only if stdint.h exists. int on the other hand is guaranteed to exist.Between int32 and int32_t, (and likewise between int8 and int8_t ) the difference is pretty simple: the C standard defines int8_t and int32_t, but does not define anything named int8 or int32 the latter (f they exist at all) is probably from some other header or library (most likely predates the addition of int8_t and int32_t in C9g). Plain int is quite a bit different from the others. Where int8_t and int32_t each have a specified size, int can be any size >= 16 bits. At different times, both 16 bits and 32 bits have been reasonably common (and for a 64-bit implementation, it should probably be 64 bits). On the other hand, int is guaranteed to be present in every implementation of C, where int8_t and int32_t are not. It’s probably open to question whether this matters to you though. If you use C on small embedded systems and/or older compilers, it may be a problem. If you use it primarily with a modern compiler on desktop/server machines, it probably won’t be. Oops missed the part about char . You’d use int8_t instead of char if (and only if) you want an integer type guaranteed to be exactly 8 bits in size. If you want to store characters, you probably want to use char instead. Its size can vary (in terms of number of bits) but it’s guaranteed to be exactly one byte. One slight oddity though: there’s no guarantee about whether a plain char is signed or unsigned (and many compilers can make it either one, depending on a compile-time flag). If you need to ensure its being either signed Or unsigned, you need to specify that explicitly.